Using Common Dialog Boxes
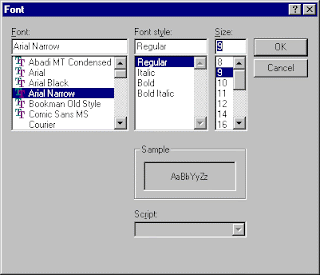
Lab 9.2: Using Common Dialog Boxes In this lab, you will add functionality to an application using the custom dialog box. Estimated time to complete this lab: 15 minutes To complete the exercises in this lab, you must have the required software. For detailed information about the labs and setup for the labs, see Labs in this course. Objectives After completing this lab, you will be able to: ® Use a common font dialog box in an application. ® Use a common color selection dialog box in an application. ® Modify the color and font in CRichEditView . Prerequisites There are no prerequisites for this lab. Exercises The following exercises provide practice with the concepts and techniques covered in this chapter. ® Exercise 1: Adding a Font Dialog Box In this exercise, you will add a font dialog box to an application. ® Exercise 2: Adding a Color Selection Dialog Box In this exercise, you will add a color-choice dialog box to an application. Exercise 1: Adding a Font Di